ZingGrid Object Methods
This is a comprehensive list of available functionality on the global window.ZingGrid
object. It is redundant to define window
, so we will call this the ZingGrid
object.
registerAdapter()
You use the registerAdapter()
method to define how to hook up a data source into your project. If you have your own standardized endpoints, this is very useful as it's a way for us to provide an ES6 style import mechanism and pattern for building custom data sources.
Check out the docs on <zg-param>
attributes to get a full list of available adapter options here.
Function Definition
ZingGrid.registerAdapter(sType, oOptions)
Name | Type | Optional | Description |
---|---|---|---|
sType | String | false | The string name for the adapter |
oOptions | Object | false |
Option list of of adapter variables you want to define. You can define ANYzg-param value here.
|
HTML before registerAdapter()
<zing-grid editor caption="Movies" page-size="10" infinite height="100px"> <zg-data src="https://zinggrid-examples.firebaseio.com/movies/"> <zg-param name="startAtKey" value="startAt"></zg-param> <zg-param name="limitToKey" value="limitToFirst"></zg-param> <zg-param name="sortByKey" value="orderBy"></zg-param> <zg-param name="searchKey" value="equalTo"></zg-param> <zg-param name="startAtValue" value="true"></zg-param> <zg-param name="addValueQuotes" value="true"></zg-param> <zg-param name="sortBy" value="title"></zg-param> </zg-data> </zing-grid>
HTML after registerAdapter()
<zing-grid editor caption="Movies" page-size="10" infinite height="100px"> <zg-data src="https://zinggrid-examples.firebaseio.com/movies/" adapter="myCustomAdapter"></zg-data> </zing-grid>
JavaScript
const adapterOptions = { limitToKey: 'limitToFirst', startAtKey: 'startAt', sortByKey: 'orderBy', startAtValue: true, sortBy: '"title"', }; ZingGrid.registerAdapter('myCustomAdapter', adapterOptions);
registerAdapter() Grid
Here is a complete grid which calls the registerAdapter()
method:
registerCellType()
You use the registerCellType()
method to create and assign a custom column type.
Function Definition
ZingGrid.registerCellType(sType, oOptions)
Name | Type | Optional | Description |
---|---|---|---|
sType | String | false | The name of the cell type you want to define |
oOptions | Object | false | An object to define the renderer and/or editor for the cell type |
HTML
<zg-column index="col" type="upper" header="College"></zg-column>
JavaScript
// renderer function for cell function upperRenderer(mRawData, cellRef, $cell) { return mRawData.toUpperCase(); } // hooks for editor let editor = { init($cell, editorField) { let oDOMInput = document.createElement('input'); oDOMInput.type = 'text'; oDOMInput.autoComplete = 'off'; oDOMInput.ariaInvalid = false; editorField.appendChild(oDOMInput); }, onOpen($cell, editorField, mData) { let oDOMInput = editorField.querySelector('input'); if (!mData) { mData = editorField.value || ''; } oDOMInput.value = String(mData); }, onClose(editorField) { return 'Edited: ' + editorField.querySelector('input').value; }, }; ZingGrid.registerCellType('upper', { editor: editor, renderer: upperRenderer, });
registerCellType() Grid
Here is a complete grid which calls the registerCellType()
method:
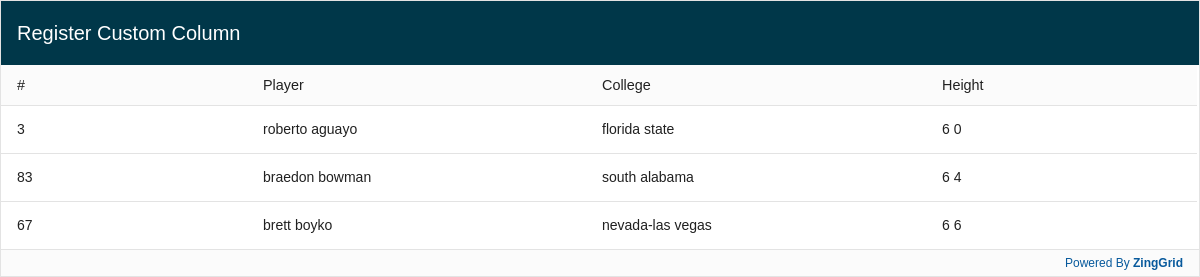
registerClient()
You use the registerClient()
method to register a third party client to make it available to ZingGrid for JS adapter calls.
Currently configured for supabaseJS adapter.
Function Definition
ZingGrid.registerClient(oClient, oScope)
Name | Type | Optional | Description |
---|---|---|---|
oClient | Object | false | The client that will be used for the adapter calls |
oScope | Object | true | Scope of the client |
HTML
<zing-grid> <zg-data adapter="supabaseJS"> <zg-param name="dataTable" value="employee_list"></zg-param> </zg-data> </zing-grid>
JavaScript
const supabaseClient = supabase.createClient(SUPABASE_URL, SUPABASE_KEY); ZingGrid.registerClient(supabaseClient);
registerClient() Grid
Here is a complete grid which calls the registerClient()
method:

registerCustomFilterMethod()
The registerCustomFilterMethod()
method registers a custom filter method. The custom filter method can then be added to the list of filter conditions that a column can be filtered by.
Function Definition
ZingGrid.registerCustomFilterMethod(key, oCustomFilterObj, oScope);
Name | Type | Optional | Description |
---|---|---|---|
key | String | false | The key of the custom filter method. This keys will be used to add to `getConditions()` or in `[filter-conditions]` |
oCustomFilterObj | Object | false | Object containing the custom filter. It contains three properties: - title: What displays in the conditions selectbox - fieldCount: Number of text fields to display for this condition (0-2) - showMatchCase: Boolean indicating if match case checkbox should be displayed. Default is true. - filterMethod: Points to the method to call when filter is run. Filter method will be passed `oConfig` object that contains: - dataVal: Value from the grid's data - [val1]: Value of first text field (if present) - [val2]: Value of second text field (if present) - matchCase: Boolean indicating if user selectd the match base checkbox |
oScope | Object | true | Scope of the filter method |
HTML
<zing-grid filter filter-conditions="customCondition, break, default">
JavaScript
let customCondition = { title: "Starts With M", fieldCount: 0, filterMethod: (oConfig) => { return oConfig.dataVal.indexOf('M') === 0; } }; ZingGrid.registerCustomFilterMethod('customCondition', customCondition);
registerCustomFilterMethod() Grid
Here is a complete grid which calls the registerCustomFilterMethod()
method:
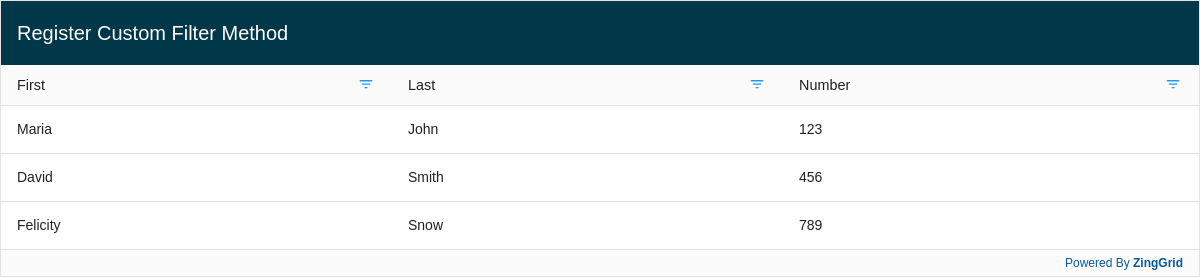
registerEditor()
The registerEditor()
method is used to create and assign a custom editor to the grid.
Function Definition
ZingGrid.registerEditor(oConfig, sName, oScope);
Name | Type | Optional | Description |
---|---|---|---|
oConfig | Object | false | The object containing editor life cycle hooks for cell editing (`init`, `onOpen`, `onClose`) |
sName | String | false | String name of the custom editor |
oScope | Object | true | Scope of the editor |
HTML
<zg-column index="col" editor="prependEdited"></zg-column>
JavaScript
// hooks for editor let editor = { init($cell, editorField) { let oDOMInput = document.createElement('input'); oDOMInput.type = 'text'; oDOMInput.autoComplete = 'off'; oDOMInput.ariaInvalid = false; editorField.appendChild(oDOMInput); }, onOpen($cell, editorField, mData) { let oDOMInput = editorField.querySelector('input'); if (!mData) { mData = editorField.value || ''; } oDOMInput.value = String(mData); }, onClose(editorField) { return 'Edited: ' + editorField.querySelector('input').value; }, }; ZingGrid.registerEditor(editor, 'prependEdited');
registerEditor() Grid
Here is a complete grid which calls the registerEditor()
method:
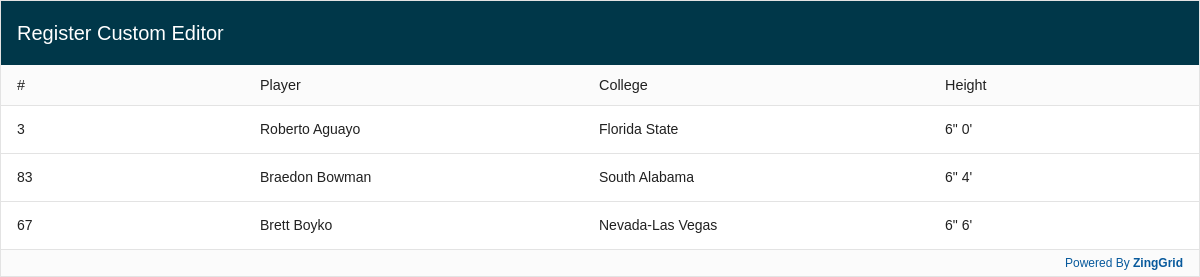
registerFilterer()
The registerFilterer()
method registers the life cycle hooks for filterer.
Function Definition
ZingGrid.registerFilterer(fnMethod, sName, oScope);
Name | Type | Optional | Description |
---|---|---|---|
oConfig | Object | false | Object containing filterer life cycle hooks (`afterInit`) |
sName | String | false | String name of the custom filterer |
oScope | Object | true | Scope of the filterer |
HTML
<zg-column index="last" filterer="lettersOnly"></zg-column>
Javascript
let customFilter = { afterInit(oDOMFilter) { let oDOMInput = oDOMFilter.querySelector('input'); oDOMInput.addEventListener('keypress', (e) => { if (!/^[a-z]$/i.test(e.key)) e.preventDefault(); }); }, }; ZingGrid.registerFilterer(customFilter, 'lettersOnly');
registerFilterer() Grid
Here is a complete grid which calls the registerFilterer()
method:
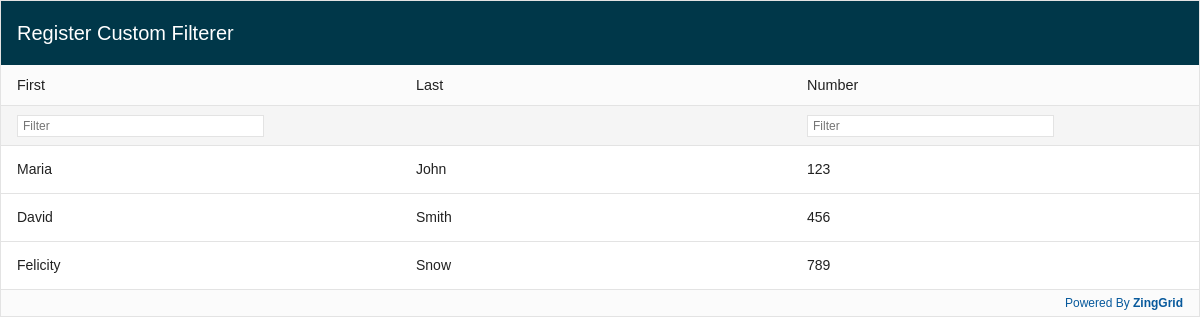
registerMethod()
You use the registerMethod()
method to assign an alias to a single method outside the window scope, which will be found and assigned a scope reference to this
.
Function Definition
ZingGrid.registerMethod(fnMethod, sName, oScope)
Name | Type | Optional | Description |
---|---|---|---|
fnMethod | Function | false | The method that you want to expose to ZingGrid |
sName | String | true | The name to refer to the method. If the method is not anonymous, the name will default to the name of the method. If it is anonymous, a name must be set. Whatever is set here is how you should refer to the method in the grid. |
oScope | Object | true |
The scope of the method. When the method is called, this will be set to the scope value.
|
HTML
<zg-column index="high" type="currency" cell-class="f1"></zg-column> <zg-column index="low" type="currency" cell-class="f2"></zg-column>
JavaScript
const namespace1 = { // global vars for highlight min/max values gHigh: -1, gLow: Number.MAX_SAFE_INTEGER, // highlight cell with highest value in high column _highlightHigh: function(high, cellDOMRef, cellRef) { if (high == Number(this.gHigh)) return 'highlight'; }, // highlight cell with lowest value in low column _highlightLow: function(low, cellDOMRef, cellRef) { if (low == Number(this.gLow)) return 'highlight'; }, }; // register methods to alias "f1" and "f2" where "this" references the // namespace1 scope as well ZingGrid.registerMethod(namespace1._highlightHigh, 'f1', namespace1); ZingGrid.registerMethod(namespace1._highlightLow, 'f2', namespace1);
registerMethod() Grid
Here is a complete grid which calls the registerMethod()
method:
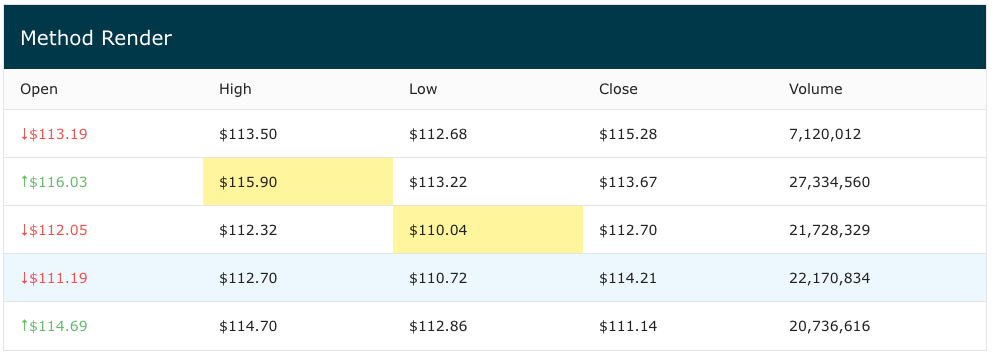
registerNamespace()
You use the registerNamespace()
method to assign a namespace alias so that all methods outside the window scope can be found, and assigned a scope reference to this
. Once a namespace is registered, the methods within the namespace will be accessible to ZingGrid without having to call ZingGrid.registerMethod
.
Function Definition
ZingGrid.registerNamespace(oNamespace, sName, oScope)
Name | Type | Optional | Description |
---|---|---|---|
oNamespace | Function | false | The namespace that you want to expose to ZingGrid |
sName | String | true | The name to reference the namespace |
oScope | Object | true | The scope of the namespace. When a method within the namespace is called, `this` will be set to the `scope` value. Defaults to the namespace itself. |
HTML
<zg-column index="low" type="currency" cell-class="n1._highlightLow"></zg-column>
JavaScript
const namespace1 = { // global vars for highlight min/max values gHigh: -1, gLow: Number.MAX_SAFE_INTEGER, // highlight cell with lowest value in low column _highlightLow: function(low, cellDOMRef, cellRef) { if (low == Number(this.gLow)) return 'highlight'; }, }; // register namespace to alias "n1" where "this" references the // namespace1 scope as well ZingGrid.registerNamespace(namespace1, 'n1', namespace1);
registerNamespace() Grid
Here is a complete grid which calls the registerNamespace()
method:
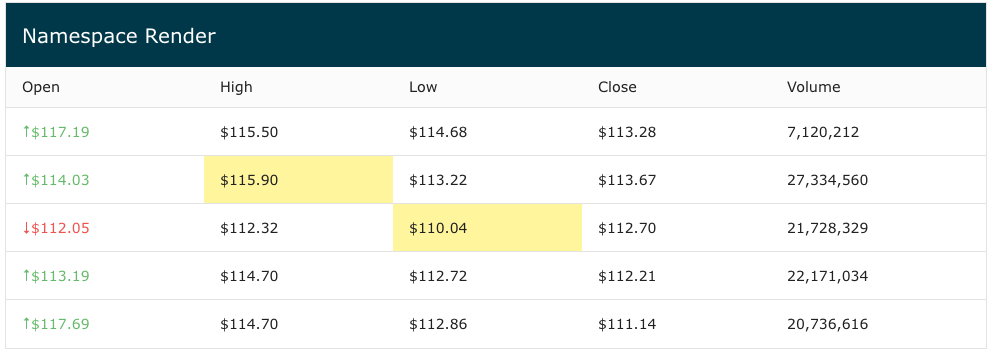
registerValidator()
To register life cycle hooks for cell validation, use registerValidator()
.
Function Defintiion
ZingGrid.registerValidator(oValidator, sName, oScope);
Name | Type | Optional | Description |
---|---|---|---|
oValidator | Object | false | Object of validator life cycle hooks to expose to the grid |
sName | String | true | The name to refer to the validator. |
oScope | Object | true | Scope of the validator |
HTML
<zg-column index="first" validator="customValidator"></zg-column>
JavaScript
let customValidator = function(text) { return text.includes('testing'); }; ZingGrid.registerValidator(customValidator, 'customValidator');
registerValidator() Grid
Here is a complete grid which calls the registerValidator()
method:
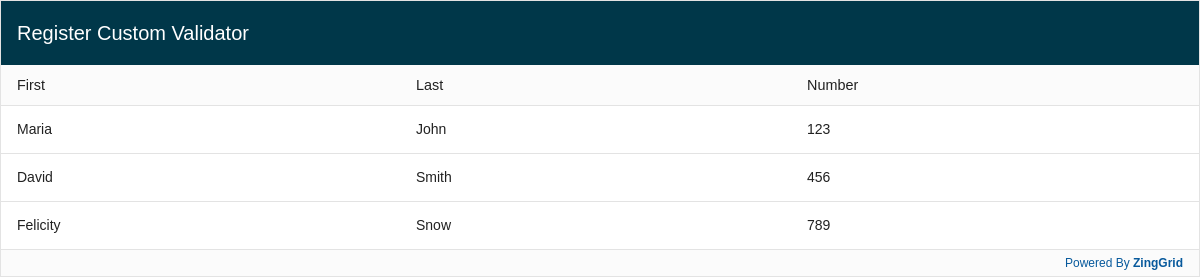
customizeDialog()
Use customizeDialog()
to customize the dialog for all instances of ZIngGrid.
Function Defintiion
ZingGrid.customizeDialog(type, config);
Name | Type | Optional | Description |
---|---|---|---|
type | String | false | Type of dialog to customize. If set to `null`, the config will apply to all dialogs. The options are: field-update, record-create, record-delete, record-info, record-update, view-error, view-info, view-success, view-warn, zg-version. |
config | Object | false | Options for data retrieval. The options are: - cancel: Text for cancel button - confirm: Text for confirm button - label: Label to display on the dialog |
HTML
<zing-grid editor="modal">
JavaScript
ZingGrid.customizeDialog('field-udpate', { cancel: 'CANCEL ACTION', confirm: 'CONFIRM ACTION', label: 'DIALOG ACTIONS' });
customizeDialog() Grid
Here is a complete grid which calls the customizeDialog()
method:
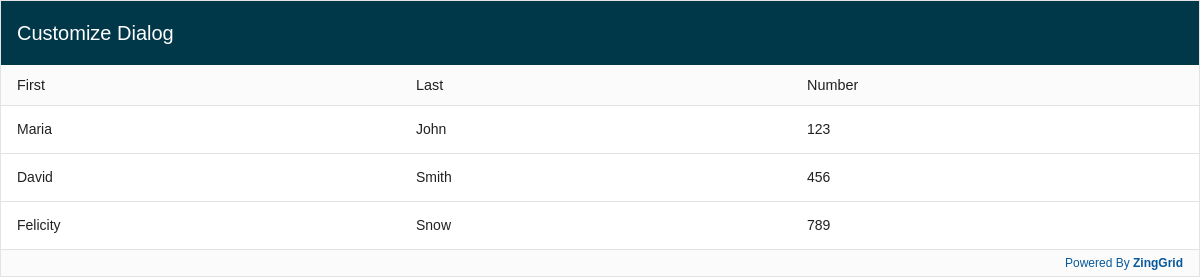
formatDate()
Use customizeDialog()
to customize the dialog for all instances of ZIngGrid.
Function Defintiion
ZingGrid.customizeDialog(type, config);
Name | Type | Optional | Description |
---|---|---|---|
type | String | false | Type of dialog to customize. If set to `null`, the config will apply to all dialogs. The options are: field-update, record-create, record-delete, record-info, record-update, view-error, view-info, view-success, view-warn, zg-version. |
config | Object | false | Options for data retrieval. The options are: - cancel: Text for cancel button - confirm: Text for confirm button - label: Label to display on the dialog |
HTML
<zing-grid editor="modal">
JavaScript
ZingGrid.customizeDialog('field-udpate', { cancel: 'CANCEL ACTION', confirm: 'CONFIRM ACTION', label: 'DIALOG ACTIONS' });
customizeDialog() Grid
Here is a complete grid which calls the customizeDialog()
method:
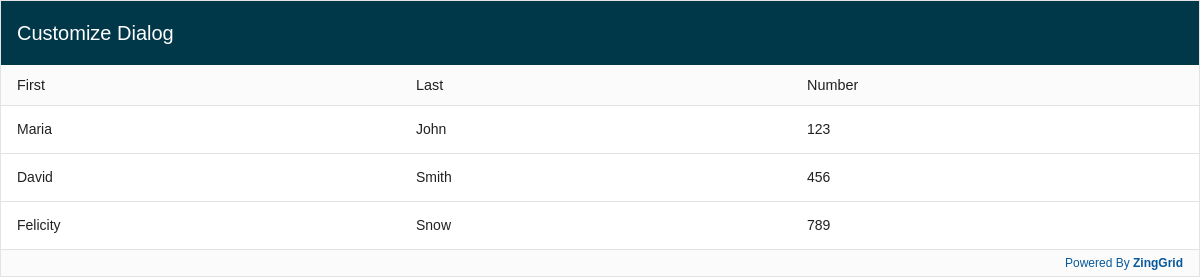
fromNow()
The fromNow()
formats a Date
object in "from now" format.
Function Defintiion
ZingGrid.fromNow(date, raw, lang);
Name | Type | Optional | Description |
---|---|---|---|
date | SDate | false | The `Date` to format |
raw | Boolean | false | Indicates if we should include "ago/to" to indicate past/future |
lang | String | true | The language to use for formatting |
HTML
<zg-column index="date" type="date" renderer="renderDate"></zg-column>
JavaScript
let renderDate = function(date) { return `${ZingGrid.fromNow(date, true)} too late`; }; ZingGrid.registerMethod(renderDate, 'renderDate');
fromNow() Grid
Here is a complete grid which calls the fromNow()
method:
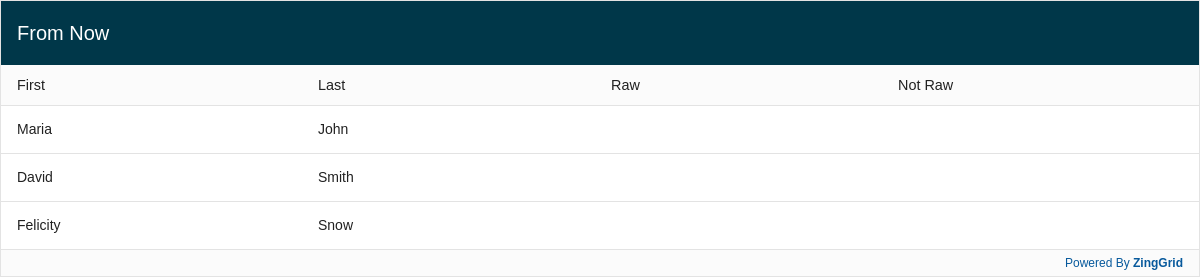
Use customizeDialog()
to customize the dialog for all instances of ZIngGrid.
Function Defintiion
ZingGrid.customizeDialog(type, config);
Name | Type | Optional | Description |
---|---|---|---|
type | String | false | Type of dialog to customize. If set to `null`, the config will apply to all dialogs. The options are: field-update, record-create, record-delete, record-info, record-update, view-error, view-info, view-success, view-warn, zg-version. |
config | Object | false | Options for data retrieval. The options are: - cancel: Text for cancel button - confirm: Text for confirm button - label: Label to display on the dialog |
HTML
<zing-grid editor="modal">
JavaScript
ZingGrid.customizeDialog('field-udpate', { cancel: 'CANCEL ACTION', confirm: 'CONFIRM ACTION', label: 'DIALOG ACTIONS' });
customizeDialog() Grid
Here is a complete grid which calls the customizeDialog()
method:
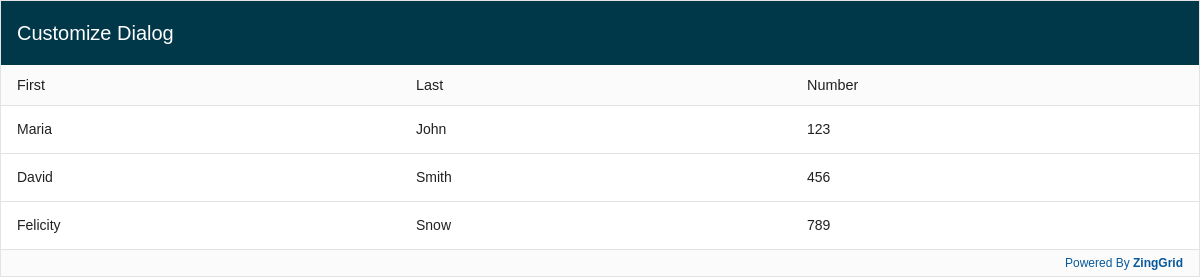
searchIsTable()
Use searchIsTable()
method to search for table element(s) with the [is="zing-grid"]
attribute to insert the <zing-grid>
. This method shoud only be used when table[is="zing-grid"]
is added after the Document
has been loaded.
Function Defintiion
ZingGrid.searchIsTable();
HTML
<table is="zing-grid"></table>
JavaScript
ZingGrid.searchIsTable();
searchIsTable() Grid
Here is a complete grid which calls the searchIsTable()
method:
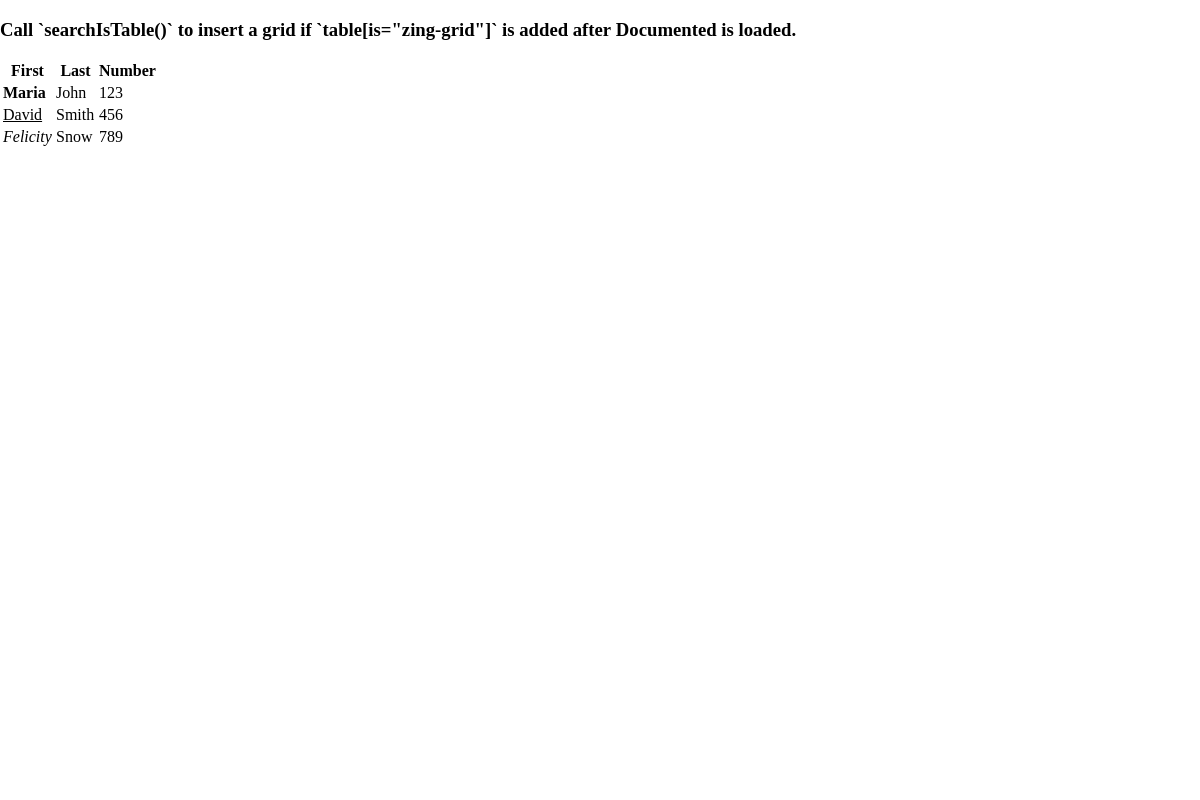
setLicense()
The setLicense()
method is used to set the license and removes the watermark is the given license is valid.
Function Defintiion
ZingGrid.setLicense(aLicense, aBuildCode);
Name | Type | Optional | Description |
---|---|---|---|
aLicense | String Array | false | List of license keys |
aBuildCode | String Array | true | List of build codes |
JavaScript
ZingGrid.setLicense(['LICENCE_KEY']);
setLicense() Grid
Here is a complete grid which calls the setLicense()
method:
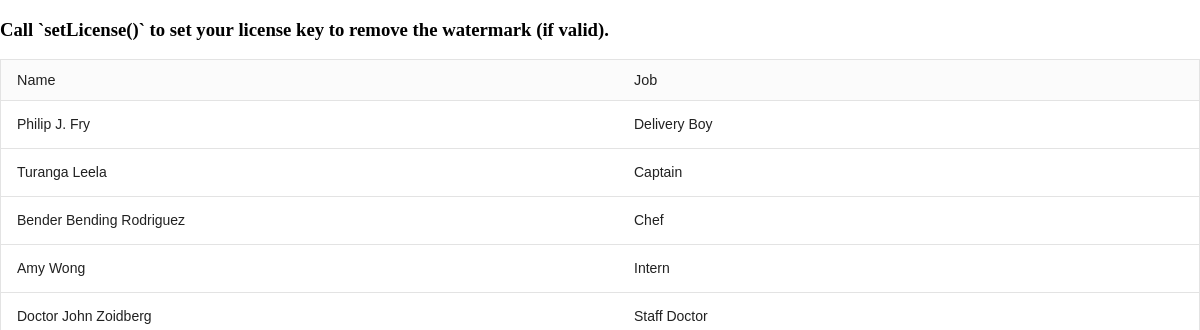
setBuildCode()
The setBuildCode()
method is used to set the build code and removes the watermark is the given build code is valid.
Function Defintiion
ZingGrid.setBuildCode(aBuildCode);
Name | Type | Optional | Description |
---|---|---|---|
aBuildCode | String Array | false | The first element of the array is the build code, and the second element is the build code id. |
JavaScript
ZingGrid.setBuildCode(['BUILD_CODE', 'BUILD_CODE_ID']);
setBuildCode() Grid
Here is a complete grid which calls the setBuildCode()
method:
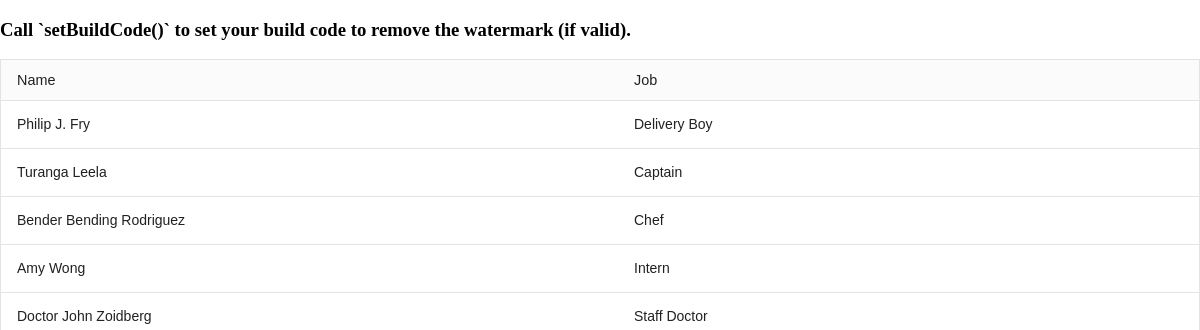
[api: ZingGrid object]