Retrieving Data from a Local REST API
In this guide, we'll walk you through how to retrieve data from a local REST API and incorporate it in your grid.
In the examples below, we use json-server as our local REST server.
For our example, our database is a JSON file that defines users
, an array of user
objects.
For our JSON file to work with json-server, our objects need to have a key called id
, which will be used when making requests to the server.
{ "users": [ { "first": "Diane", "last": "Murphy", "email": "dmurph13@gmail.com", "id": "0" }, { "first": "Gerald", "last": "Patterson", "email": "gpat@yahoo.com", "id": "1" }, ... ] }
Starting a JSON Server
From the command line, we'll start a JSON server to access our database, like so:
json-server --watch users.json
Then, we can get the path to our database. In our case, our path is: "http://localhost:3000/users/". We'll set the src
attribute of the <zing-grid>
tag to this path, like so:
<zing-grid src="http://localhost:3000/users/"></zing-grid>
REST API Data Grid
Here is our complete grid pulling in data from our database:
CRUD Grid
Setting the source to a REST API route allows us to have a simple CRUD grid. You can create new rows, read from the given source, update cells or rows, and delete rows from the grid. Any changes to the grid will automatically be reflected in the database.
You'll need to specify the id key in order for your requests to work properly. To do so, first include the <zg-data>
element. Then, set the src
attribute to the source of data and idKey
to the key used to identify each row.
To update cells/rows, you need to set the editor
attribute for the grid. You can create/update/delete rows through the context menu which appears after setting the context-menu
attribute for the grid. You can also do this by using specific column types.
Altogether, it'll look like this:
<zing-grid editor context-menu> <zg-data src="http://localhost:3000/users/" idKey="id"></zg-data> </zing-grid>
Infinite Scroll
To enable infinite scroll, you'll first need to set the infinite
attribute for your grid. Then, set the limitToKey
and pageKey
properties in the options
attribute for <zg-data>
(see HTML and demo below).
limitToKey
is the query parameter that is used to specify the max amount of records to retrieve. If you look at the paginate section of the json-server README, you will see that "_limit" is used as the query parameter; so, you'll need to set limitToKey
to "_limit". pageKey
is the query parameter used to specify the page. Similarly, you'll see that this should be set to "_page".
<zing-grid infinite> <zg-data src="http://localhost:3000/users/" options='{ "limitToKey": "_limit", "pageKey": "_page" }'> </zg-data> </zing-grid>
Infinite Scroll Enabled Grid
Here is our complete grid with infinite scroll enabled:
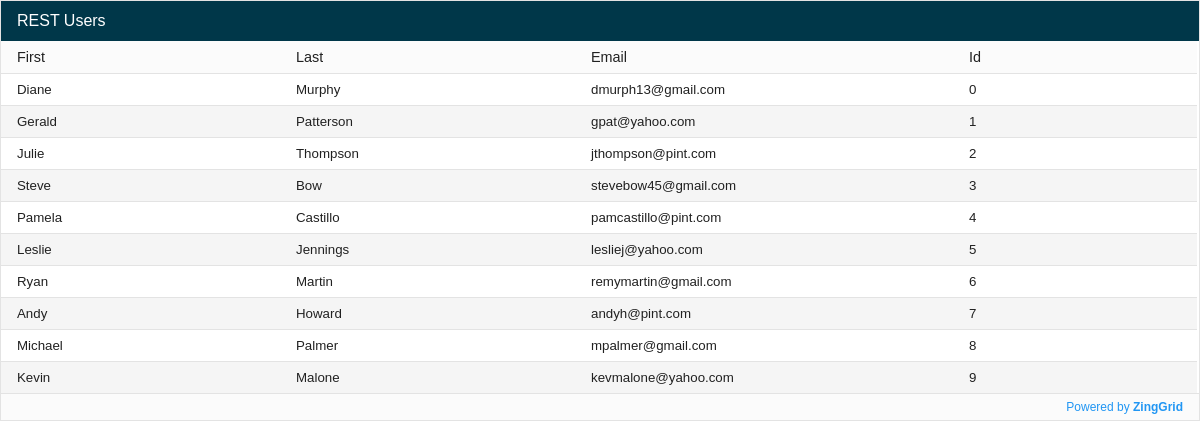
Sorting
In order to sort the data, you need to set the parameters sortByKey
, sortBy
, sortDirKey
, and sortDir
.
sortByKey
is the query parameter for sortingsortBy
is the key that we want to sort onsortDirKey
is the query parameter for the sorting directionsortDir
is the sort direction
Looking at the sort section of the json-server README, you'll see that sortByKey
should be set to "_sort" and sortDirKey
should be set to "_order".
In the example below, we will sort the data by the "first" property, and we will set the direction to be descending (Z-A):
<zing-grid> <zg-data src="http://localhost:3000/users/" options='{ "sortByKey": "_sort", "sortBy": "first", "sortDirKey": "_order", "sortDir": "desc" }'> </zg-data> </zing-grid>
Sorting Enabled Grid
Here is our complete grid which sorts the "first" property in descending (Z-A) order:
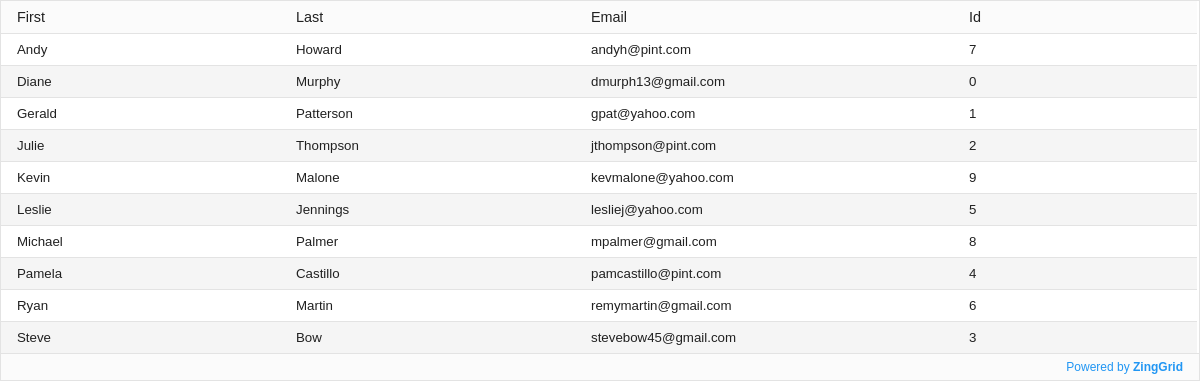
Searching
To enable server search, simply include the searchKey
attribute, like so:
<zg-param name="searchKey" value="search"></zg-param>
Searching Enabled Grid
Here is our complete grid with server search enabled:
Another Version: <zg-param>
In the examples above, most of our configurations are set in the options
object. Another way that you can write this is with the <zg-param>
tag. <zg-param>
is a child of <zg-data>
, and it has two attributes: name
and value
. Using <zg-param>
provides greater readability and allows us to focus on a more component-based approach.
Below, we have rewritten the sorting example:
<zing-grid> <zg-data> <zg-param name="sortByKey" value="_sort"></zg-param> <zg-param name="sortBy" value="first"></zg-param> <zg-param name="sortDirKey" value="_order"></zg-param> <zg-param name="sortDir" value="desc"></zg-param> <zg-param name="src" value="http://localhost:3000/users/"></zg-param> </zg-data> </zing-grid>
[data: rest]